Message queues are especially useful for debugging networking events and other function calls that may occur out of expected order.
Note: This is an advanced tutorial, recommended for those already familiar with the Unity3D game engine.
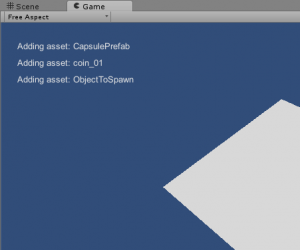
Goal:
Allow developers to see important debugging information, in-game. We also want to be able to add these debug messages from any other game script. The script should allow the programmer to define how long to the message remains in the queue (in seconds).
For example:
messageQueue.addMessage ("Adding asset: " + o.name,3);
Step-by-Step:
- First, we need to define the message itself. In this case, we’ll create a class, cMessage, that includes both a string for the message and float for the time-stamp indicating when to remove the message from the queue.
public class cMessage { public float m_exitTime; public string m_message; public cMessage(string p_msg, float p_exitTime) { m_message = p_msg; m_exitTime = p_exitTime; } }
- For the main class, we’ll use a C# List for the queue. We can also expose a few public variables defining where the queue is to be displayed on the screen as well as a flag to enable/disable the visual display.
using UnityEngine; using System.Collections; using System.Collections.Generic; //Needed for C# List<T> public class messageQueue : MonoBehaviour { private static List<cMessage> m_queue = new List<cMessage>(); private bool m_enabled = true; public Vector2 queueLocation = new Vector2(25, 25); //in pixels public Vector2 messageSize = new Vector2(200, 25); //in pixels //.. }
- To add messages to the queue, we’ll expose a static function, “addMessage”. As a static function, this can be called using the class name.
public static void addMessage(string pMessage, float pDuration) { cMessagetempMessage = new cMessage(pMessage, Time.time + pDuration); m_queue.Add(tempMessage); }
- Now, we can add the visualization of the queue within the OnGUI() function.
void OnGUI() { if (!m_enabled) return; float yPos = queueLocation.y; foreach (cMessage m in m_queue) { GUI.Label(new Rect(queueLocation.x, yPos, width, height), m.m_message); yPos+=height; } }
- Finally, add functionality to the Update() function that removes items from the queue when they have expired and allows the player to toggle the display of the queue.
void Update () { for (int i = m_queue.Count - 1; i>=0; i--) { if (Time.time > m_queue[i].m_exitTime) m_queue.RemoveAt(i); } if (Input.GetButtonDown("Fire2")) m_enabled = !m_enabled; }
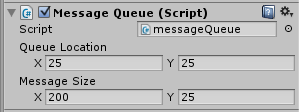
Using our messageQueue script:
Since this script inherits from MonoBehavior, it must be added to a game object within Unity. This will expose the public variables in the inspector.
Now, simply call the addMessage() function whenever/wherever needed. This is especially useful for events and loading assets.
Possible next step:
- add a priority flag (high, medium, low) that determines the color and duration a message is displayed
- include a call to Debug.Log() or your own debug file
Full source is available on GitHub.
1 comment on “Unity | Message Queue”